Create Taxonomy Term Meta in WordPress
Just like post meta data term meta is additional information stored against a taxonomy terms. A single entry in a custom taxonomy is called term. This post demonstrates how can you add term meta to your custom taxonomy. We will create a term meta "size" for our property_type taxonomy.
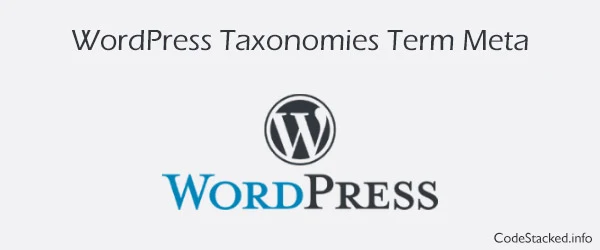
As WordPress allows us to add our custom post types and custom taxonomies, it also provides different hooks to extend functionality as per our need. For adding custom term meta we will need to use four hooks, One for showing term meta field on add term form, Second for showing term meta field on term edit form and third and fourth one to save the term meta whenever a term is created or updated. WordPress hooks can be used like this:
add_action( "$taxonomy_add_form_fields", "function_to_show_fields", 10, 1 ); add_action( "$taxonomy_edit_form_fields", "function_to_show_fields", 10, 2 ); add_action( "created_$taxonomy", "funtion_to_save_fields", 10, 2 ); add_action( "edited_$taxonomy", "funtion_to_save_fields", 10, 2 );
Where $taxonomy is the slug of taxonomy in this post its going to be size. You need to change it to your taxonomy slug for which you are going create this custom term meta. Now lets break it down in sections. We will create a separate file terms-meta.php, which will hold the following hooks code for creating our term meta.
terms-meta.php
<?php // Hook to show term field on add term form
add_action('property_type_add_form_fields', 'add_property_type_meta_fields', 10, 1);
function add_property_type_meta_fields( $taxonomy ) {?>
<div class="form-field property-type-metabox">
<label class="title-label"><?php _e('Size', 'text-domain'); ?></label>
<label class="field-radio"><input type="radio" name="size" value="small" checked="checked"><?php _e('Small', 'text-domain')?></label>
<label class="field-radio"><input type="radio" name="size" value="medium"><?php _e('Medium', 'text-domain' )?></label>
<label class="field-radio"><input type="radio" name="size" value="large"><?php _e('Large', 'text-domain' )?></label>
</div>
<?php
}
// Hook to show term fields on edit taxonomy form
add_action('property_type_edit_form_fields', 'taxonomy_property_type_edit_meta_field', 10, 2);
function taxonomy_property_type_edit_meta_field( $term, $taxonomy ) {
$size = get_term_meta( $term->term_id, 'size', true );?>
<tr class="form-field">
<th scope="row" valign="top"><?php _e('Size', 'text-domain'); ?></th>
<td>
<div class="property-type-metabox">
<label class="field-radio">
<input type="radio" name="size" value="small"
<?php _e($size == 'small' ? 'checked="checked"' : '', 'text-domain');?> />
<?php _e('Small', 'text-domain')?>
</label>
<label class="field-radio">
<input type="radio" name="size" value="medium"
<?php _e($size == 'medium' ? 'checked="checked"' : '', 'text-domain');?> />
<?php _e('Medium', 'text-domain')?>
</label>
<label class="field-radio">
<input type="radio" name="size" value="large"
<?php _e($size == 'large' ? 'checked="checked"' : '', 'text-domain');?> />
<?php _e('Large', 'text-domain')?>
</label>
</div>
</td>
</tr>
<?php
}
// Two hooks with same function to save term meta when term is created/edited
add_action('created_property_type', 'add_edit_property_type_custom_meta', 10, 2);
add_action('edited_property_type', 'add_edit_property_type_custom_meta', 10, 2);
function add_edit_property_type_custom_meta( $term_id, $tax_term_id ) {
if (isset( $_POST['size'])) {
update_term_meta( $term_id, 'size', esc_attr($_POST['size']) );
}
}
Now include "terms-meta.php" in our "functions.php"
<?php include('terms-meta.php');?>
Function used to get term meta of a single term:
get_term_meta( $term_id, 'meta_key', true );
Where $term_id is the single term id , "meta_key" is the field name we saved which was "size" used in this post, and last parameter is used for response if set to true it will return the value.
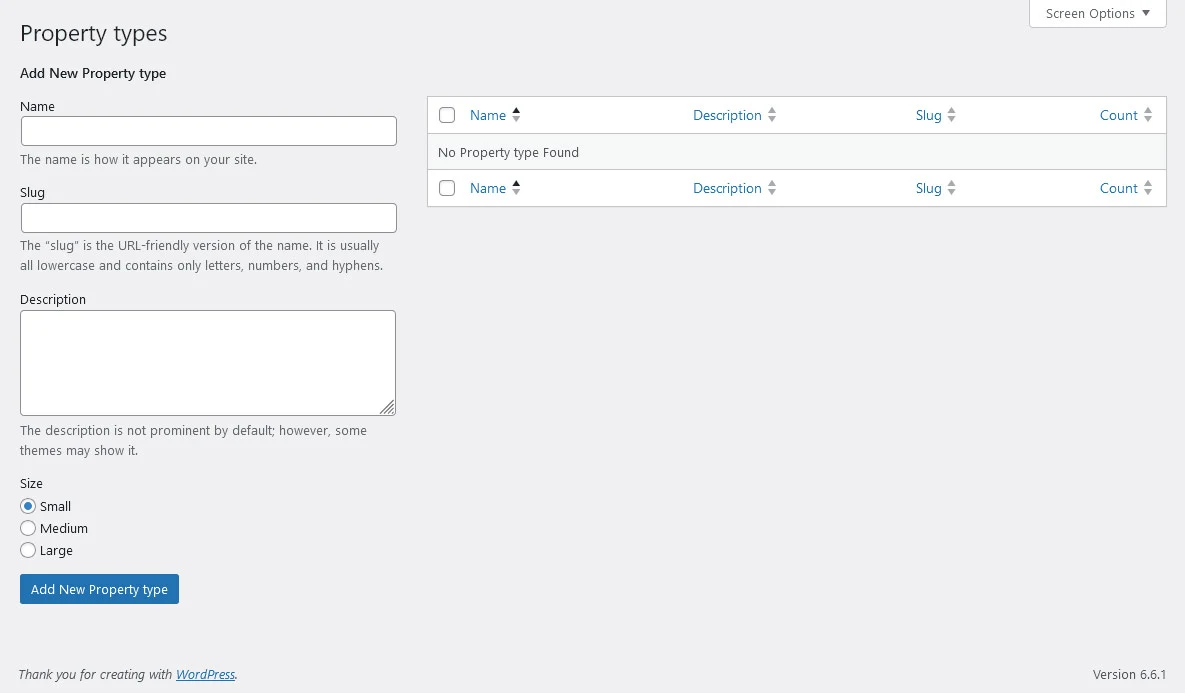